C Program For Making Apache Config Files
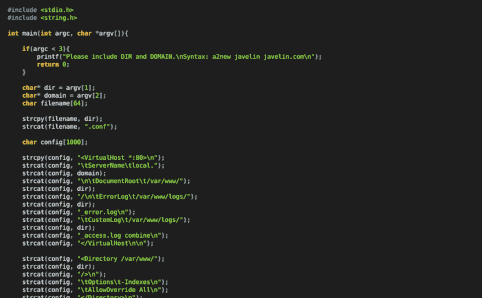
In a dev environment, Aaron likes to closely simulate domain structures while working on web projects. This is especially helpful
when using the mod_rewrite plugin. In order to use local domains, you must setup Virtual Hosts in Apache and this can become
tedious when you work on a lot of projects. This program easily creates an Apache config file based on a standard dev environment.
#include <stdio.h>
#include <string.h>
int main(int argc, char *argv[]){
if(argc < 3){
printf("Please include DIR and DOMAIN.\nSyntax: a2new javelin javelin.com\n");
return 0;
}
char* dir = argv[1];
char* domain = argv[2];
char filename[64];
strcpy(filename, dir);
strcat(filename, ".conf");
char config[1000];
strcpy(config, "<VirtualHost *:80>\n");
strcat(config, "\tServerName\tlocal.");
strcat(config, domain);
strcat(config, "\n\tDocumentRoot\t/var/www/");
strcat(config, dir);
strcat(config, "/\n\tErrorLog\t/var/www/logs/");
strcat(config, dir);
strcat(config, "_error.log\n");
strcat(config, "\tCustomLog\t/var/www/logs/");
strcat(config, dir);
strcat(config, "_access.log combine\n");
strcat(config, "</VirtualHost\n\n");
strcat(config, "<Directory /var/www/");
strcat(config, dir);
strcat(config, "/>\n");
strcat(config, "\tOptions\t-Indexes\n");
strcat(config, "\tAllowOverride All\n");
strcat(config, "</Directory>\n");
FILE *file;
file = fopen(filename, "w+");
fprintf(file, config);
fclose(file);
}